1056. Confusing Number
Easy
A confusing number is a number that when rotated 180 degrees becomes a different number with each digit valid.
We can rotate digits of a number by 180 degrees to form new digits.
- When 0, 1, 6, 8, and 9 are rotated 180 degrees, they become 0, 1, 9, 8, and 6 respectively.
- When 2, 3, 4, 5, and 7 are rotated 180 degrees, they become invalid.
Note that after rotating a number, we can ignore leading zeros.
- For example, after rotating 8000, we have 0008 which is considered as just 8.
Given an integer n, return true if it is a confusing number, or false otherwise.
Example 1:
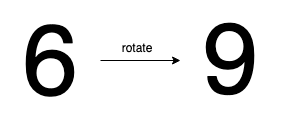
Input: n = 6
Output: true
Explanation: We get 9 after rotating 6, 9 is a valid number, and 9 != 6.
Example 2:
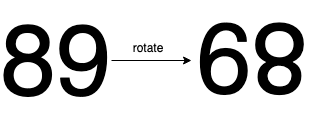
Input: n = 89
Output: true
Explanation: We get 68 after rotating 89, 68 is a valid number and 68 != 89.
Example 3:

Input: n = 11
Output: false
Explanation: We get 11 after rotating 11, 11 is a valid number but the value remains the same, thus 11 is not a confusing number
Constraints:
- 0 <= n <= 109
문제 풀이
정수 숫자가 주어지고 이 숫자들을 180도 뒤집어야한다.
0, 1, 6, 8, 9 들은 뒤집으면 유효한 숫자로 바꿀수 있다.
하지만 다른 2, 3, 4, 5, 7은 유효하지 않은 숫자로 바뀐다.
숫자를 180도 뒤집었을때 유효한 숫자이면서 주어진 숫자랑 다르면 True이고 아니면 False를 리턴한다.
딕셔너리에 키와 벨류로 뒤집어질 숫자를 미리 하드코딩한다.
n을 변경하면서 while을 진행하기 때문에 임시로 정답을 저장한다.
먼저 정답 숫자에 10을 곱한다.
주어진 숫자 n을 10으로 나머지를 구하고 그 숫자가 딕셔너리에 숫자가 있다면 정답 숫자에 더해준다.
그리고 n을 10으로 나누고 이 과정을 반복하여 n이 0이 될까지 반복한다.
계산한 값과 임시 정답을 비교하여 반환한다.
소스코드
class Solution:
def confusingNumber(self, n: int) -> bool:
rotated_dict = {
0:0,
1:1,
6:9,
8:8,
9:6
}
ans = 0
temp_n = n
while n:
temp = n % 10
if temp in rotated_dict:
ans *= 10
ans += rotated_dict[temp]
else:
return False
n //= 10
return ans != temp_n
'컴퓨터공학 > LeetCode 1000' 카테고리의 다른 글
[LeetCode] 567. Permutation in String (0) | 2023.02.10 |
---|---|
[LeetCode] 6. Zigzag Conversion (1) | 2023.02.04 |
[LeetCode] 834. Sum of Distances in Tree (0) | 2022.12.22 |
[LeetCode] 886. Possible Bipartition (0) | 2022.12.22 |
[LeetCode] 1971. Find if Path Exists in Graph (0) | 2022.12.21 |